Get amazing AI audio voiceovers made for long-form content such as podcasts, presentations and social media. (Get started for free)
Streamlining Audio Processing Leveraging Async/Await in JavaScript for Efficient Voice Cloning Workflows
Streamlining Audio Processing Leveraging Async/Await in JavaScript for Efficient Voice Cloning Workflows - Asynchronous Audio Parsing with Web Audio API
The Web Audio API offers a powerful way to handle audio within web applications, especially when dealing with complex tasks like voice cloning or creating podcasts. Its core strength lies in the ability to represent audio processing as a graph of connected nodes. This structure provides a modular and intuitive way to manipulate audio, leading to more flexible and adaptable workflows.
One crucial aspect of this API is the potential for asynchronous operations. Employing asynchronous techniques, such as those provided by async/await, is critical for smooth audio handling. Synchronous processing, while seemingly simpler, can introduce significant delays and stutters into the audio stream. In contrast, the asynchronous approach avoids blocking the main thread, ensuring that the user experience remains fluid even when the audio processing is computationally intensive.
Furthermore, the Web Audio API's capability to manage diverse audio formats, such as WAV, MP3, and AAC, expands its usefulness. This feature coupled with asynchronous loading can create robust and responsive applications that can readily process a variety of audio content. Developers can manage the loading of these files without worrying about interrupting the main thread, ultimately leading to a superior user experience. Although there are some format limitations, which depend on the browser, the API offers a flexible pathway to working with a vast range of audio material.
While handling demanding tasks, maintaining precise timing and adjusting for processing lags is crucial, especially to avoid introducing noticeable audio glitches. These considerations are more critical in applications like voice cloning where subtle inaccuracies could lead to degraded quality or a sense of artificiality. In the grand scheme of these complex audio processing tasks, this asynchronous handling approach, combined with careful attention to timing nuances, enhances the overall user experience by producing smooth and responsive audio manipulation, crucial in projects that involve intricate voice and audio processing like the ones discussed.
The Web Audio API provides a powerful, yet sometimes quirky, way to interact with sound within web applications. It enables fine-grained manipulation of audio by organizing operations within an audio context, essentially a graph built from interconnected processing units called audio nodes. These nodes allow for flexible routing and modification of audio signals, facilitating a wide range of audio manipulation tasks. While the API supports various audio formats like WAV and MP3, browser compatibility can be a bit of a headache.
Storing audio for processing involves the use of audio buffers, which are suitable for relatively short to medium-length sounds. Loading these buffers relies on techniques like XMLHttpRequest to fetch audio file data from external sources, which, when not implemented carefully, can introduce complexities, especially in managing asynchronous operations. The API offers a toolkit for more advanced applications too, like creating immersive audio experiences through spatial sound, adding real-time effects, and even visualizing audio data in real-time.
Async/await is key to improving how we work with audio. Asynchronous audio parsing within the API leverages the efficiency of JavaScript's async/await patterns, which is a massive help in dealing with large amounts of audio data in applications like voice cloning, preventing blocking and keeping audio smooth. Synchronous operations, on the other hand, can introduce delays that affect playback quality. However, we still need to be mindful of the interplay between audio timing, especially when the CPU is overloaded, as even with async handling, maintaining proper alignment of audio clips to avoid stuttering remains important.
The ability to integrate various audio sources and effects means that the API offers significant flexibility. While this provides great power, it can be complex to manage. We can weave together various audio sources, apply effects, and manage a complex audio workflow all from a relatively easy-to-use interface. It's worth noting that depending on the specifics of the algorithm and implementation, the complexity of audio manipulations might result in unexpected limitations or behaviors.
One area of interest is the API's ability to facilitate "zero-copy" buffering, which promises to boost performance by eliminating unnecessary data copies when we're processing audio. This can be very helpful for tasks like voice cloning. The ability to finely control how audio nodes are linked is a powerful aspect, allowing us to construct complicated audio graphs that can efficiently handle multiple voice streams, like when dealing with podcasts or audiobook production. The built-in high-resolution timer lets us fine-tune timing, which is crucial for synchronizing, say, cloned voices with accompanying text or other audio segments.
We can also examine real-time analysis of audio streams. Async audio parsing makes it possible to do this, providing immediate feedback during voice cloning or production that can be helpful in optimizing the final output. A crucial aspect is that the Web Audio API is a cross-platform solution, leading to a standardized experience across diverse devices for things like audiobooks and podcasts. This API lets us dynamically apply audio effects while audio is being processed, like adding reverb or equalization, and does it in a way that mitigates latency issues.
The async/await approach encourages an event-driven structure in JavaScript applications, which improves how we manage the flow of audio data. This means audio processing is unlikely to bog down the main application. Using the MediaDevices interface, we can integrate user audio input to create interactive features where users can submit voices for cloning or interact with audiobooks in real time. By relying on asynchronous operations, we are better equipped to handle and minimize latency in audio applications, which is crucial for maintaining a natural flow in applications like voice interactions or voice cloning projects. WebAssembly can also be included with the API to support more intense processing tasks. This means the API can integrate with more complex algorithms that could enhance voice cloning and the general audio experience on web platforms.
Streamlining Audio Processing Leveraging Async/Await in JavaScript for Efficient Voice Cloning Workflows - Implementing AudioWorklet for Off-Main-Thread Processing
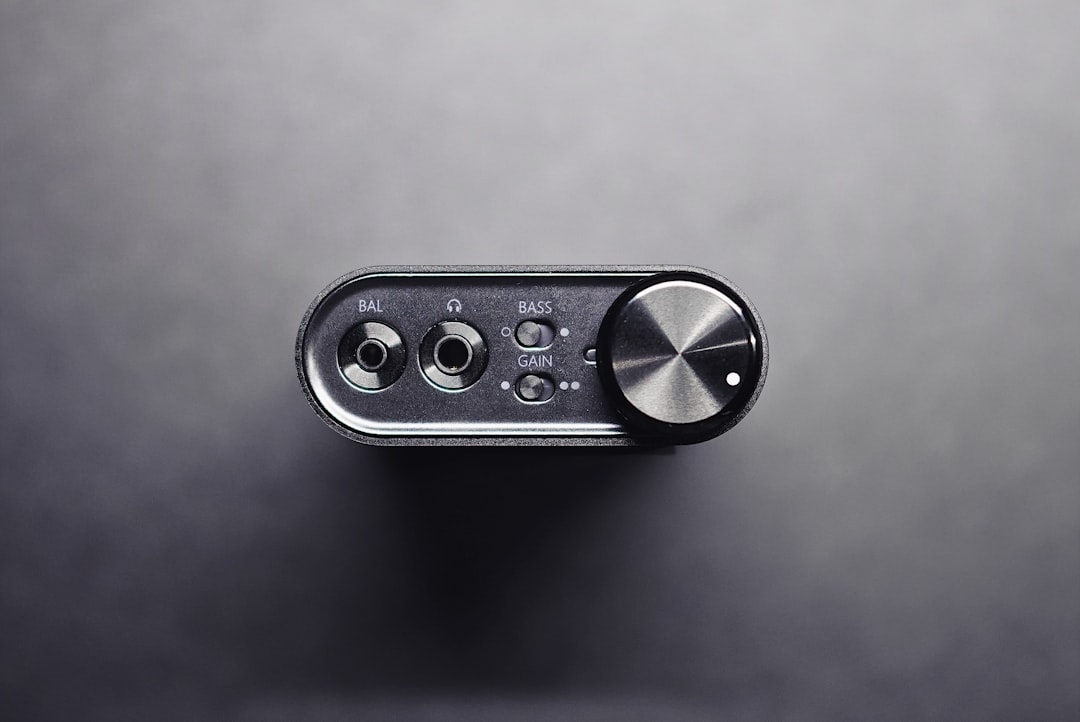
Offloading demanding audio processing tasks from the main browser thread is achievable through the implementation of AudioWorklet. This approach, which leverages a separate thread for audio processing, helps minimize latency and prevents disruptions to the user experience caused by other browser processes. This is especially advantageous in applications where audio fidelity and real-time responsiveness are critical, such as voice cloning, audiobook production, or podcast creation.
The core of AudioWorklet lies in custom audio processors built using JavaScript. By encapsulating the audio processing logic within a class derived from `AudioWorkletProcessor`, developers can craft bespoke solutions tailored for specific audio manipulations. These processors are then loaded into the audio context using the `addModule` method, thus establishing a dedicated processing pipeline.
While older techniques like `ScriptProcessorNode` were less efficient due to their reliance on the main thread, AudioWorklet's design provides a cleaner, more robust solution, particularly when integrating asynchronous operations like those made possible with async/await. This feature is valuable for optimizing intensive workflows such as voice cloning, where smoother operations and improved efficiency are crucial. In essence, AudioWorklet represents a substantial improvement for high-performance audio applications, enabling sophisticated real-time processing capabilities in a web environment previously restricted to desktop platforms. This improvement, however, might be somewhat limited due to certain browser inconsistencies or security limitations which developers need to be aware of.
AudioWorklet, a component of the Web Audio API, is a way to create custom audio processing routines in JavaScript that run outside the main browser thread. This offloading is pretty significant for things like voice cloning where low latency is critical. Even tiny delays can throw off the natural flow of a voice and ruin the illusion of a seamless clone.
The beauty of running the audio processing in a separate thread is that it doesn't interfere with other browser tasks. This means the main thread isn't bogged down when we're manipulating audio, and it keeps the user interface responsive, a big plus for interactive audio applications. The process of implementing an AudioWorklet involves using the `audioWorklet.addModule` method on the audio context to load a separate JavaScript file where the audio processor's logic resides.
One thing I like is how it avoids a problem that plagued the older `ScriptProcessorNode`, namely the issues related to asynchronous event handling and tying things to the main thread. In AudioWorklet, the audio processing is done within a dedicated class that extends `AudioWorkletProcessor`. The heart of the processing is handled within the `process` method. It all happens in its own dedicated thread built for real-time audio processing, which helps keep things running smoothly.
This separation gives us the possibility of running complex audio processing logic, like the kinds needed for high-performance audio software you'd find on a desktop, in a web browser. The code for the worklet operates within its own dedicated environment called `AudioWorkletGlobalScope`. It can even access shared resources like `SharedArrayBuffer` and `Atomics`. However, we must keep in mind that some security measures recently added in browsers require user interaction before you can create an `AudioContext` -- not a big hurdle, but something to be aware of.
The potential here for streamlining advanced audio techniques, such as voice cloning, is very interesting. Integrating async/await really smooths out the operations. We can create some quite complex audio workflows thanks to this capability.
Of course, while the potential is there, sometimes the implementation can be a bit tricky, so experimentation and troubleshooting are common. But in the big picture, we see that this is a pretty solid choice if we're creating audio tools on the web. The AudioWorklet seems quite future-proof, as web technologies mature and new audio-related features appear, and it's likely to become even more useful for tasks such as sophisticated voice manipulation.
Streamlining Audio Processing Leveraging Async/Await in JavaScript for Efficient Voice Cloning Workflows - AWS S3 Integration for Automated Voice Transcription
Integrating AWS S3 with automated voice transcription services provides a powerful way to manage and process audio data, which is especially relevant for applications like voice cloning, audiobook creation, and podcasting. AWS S3 offers a reliable and scalable storage solution for audio files, making it ideal for handling large volumes of data associated with these tasks. Amazon Transcribe, an AWS service that automatically converts speech to text, can be paired with S3 to build a streamlined transcription pipeline. This combination simplifies the process of getting text from audio, enabling more efficient workflows for projects involving large audio datasets.
The ability to handle both batch and real-time transcriptions through the integration of S3 and Transcribe adds to the flexibility of the system. For instance, pre-recorded audio can be easily processed in batches, while live audio streams can be transcribed in real time. The combination also works well with AWS Lambda, enabling automated transcription processes. Essentially, Lambda can be set up to trigger the transcription process as soon as new audio files are uploaded to S3, automating a significant portion of the workflow. This integration offers the possibility of improving efficiency and reducing the manual effort required for audio transcription in various sound-related projects. While this automation is a positive development, it's crucial to remember that relying solely on automatic speech recognition can sometimes lead to inaccuracies, especially with complex audio or unique voices, requiring developers to potentially incorporate quality control measures to ensure acceptable output quality. Overall, AWS's approach presents a powerful platform for building sophisticated audio processing solutions, though careful consideration is needed to avoid blindly accepting the outputs without appropriate review in specific contexts.
AWS S3 offers a compelling solution for storing and managing audio data within audio production workflows, particularly for tasks like voice cloning, podcasting, and audiobook creation. Its strength lies in its ability to provide secure and scalable storage, which is essential for handling potentially large volumes of audio files. One interesting aspect is how quickly data can be retrieved, especially when using regional S3 buckets. This reduces latency, which is crucial for applications needing swift audio processing, like real-time podcasting or audio book streaming.
Amazon Transcribe is a valuable AWS service that simplifies integrating automatic speech recognition (ASR) capabilities into applications. It's a fully managed service, which means you don't have to worry about infrastructure or maintaining complex speech-to-text models. Leveraging a next-generation speech foundation model, it boasts high accuracy in transcribing both live and recorded audio, making it a suitable choice for projects needing reliable transcriptions. Interestingly, the combination of S3 and Transcribe provides a good basis for constructing scalable audio transcription pipelines. This is further enhanced when used with AWS Lambda, making the process quite flexible and automated.
While Transcribe excels in providing batch and real-time transcriptions, it's also capable of processing pre-recorded media via streaming, which makes it versatile for a variety of audio processing tasks. The AWS Management Console allows you to easily set up transcription jobs. You specify where the audio files are in your S3 bucket and where the resulting transcription should be stored (also within S3). There's a Free Tier offered, which provides 60 minutes of transcription service per month for 12 months, allowing developers and researchers to experiment and gain initial experience with the platform before committing to a paid tier.
The seamless integration of AWS services makes audio transcription workflows highly automated. Using AWS Lambda, events within S3, like a new audio file being uploaded, can trigger automated actions, such as starting a voice transcription. This can simplify and expedite the workflow. While AWS S3 and Transcribe are the core duo, it's worth noting that integrating other AWS services can enhance these workflows further. Amazon Connect, Kinesis Video Streams, and DynamoDB, for example, can add complexity, but also offer additional capabilities to more elaborate audio processing chains.
Expanding on automation, services like Amazon Bedrock, which leverages generative AI models, could help with summarizing the transcriptions in a concise format. However, we need to be careful about the trustworthiness and factual integrity of the summaries produced by AI systems, as they can sometimes be unreliable. Ultimately, leveraging the S3-Transcribe-Lambda integration allows for cost-effective and scalable audio processing and transcription pipelines, particularly in audio applications that deal with a high volume of audio files like audiobooks or voice cloning projects. It's worth noting that, despite its strengths, S3-based workflows also need careful monitoring, especially related to pricing, security, and data handling as they can quickly become expensive or prone to errors if not properly managed.
Streamlining Audio Processing Leveraging Async/Await in JavaScript for Efficient Voice Cloning Workflows - Lambda Functions Triggering Audio File Workflows
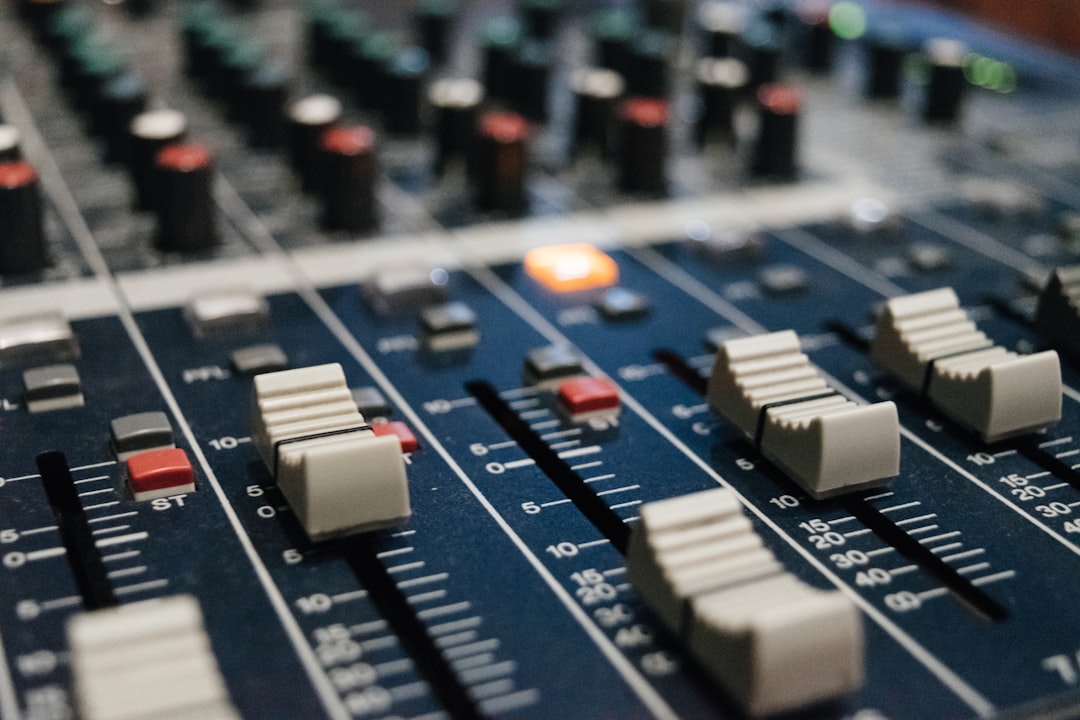
Lambda functions can trigger automated workflows for audio files, particularly when integrated with services like S3. When a new audio file is uploaded to an S3 bucket, it can activate a Lambda function, starting tasks like audio transcription or other audio manipulation. This seamless integration streamlines workflows, enhancing the efficiency of tasks related to sound production, voice cloning, or podcasting.
The power of Lambda comes from its asynchronous execution capability. Combined with JavaScript's async/await construct, it allows for smooth audio processing without blocking the main application. This is especially crucial in computationally demanding processes found in, for example, synthesizing realistic cloned voices. Complex processing chains can also be managed using AWS Step Functions, which coordinate sequences of Lambda functions. This orchestration feature is useful in scenarios like audiobook production, where multiple steps are needed to process vast audio datasets.
However, the advantages of automated workflows should be balanced with considerations for accuracy and quality. Automatic audio processing can introduce errors, especially when dealing with unique voices or complex audio segments. Developers must incorporate checks and balances to ensure the output meets quality standards. While automated processing using Lambda functions can dramatically improve efficiency, the trade-off in potential errors must be considered and addressed.
Lambda functions, triggered by events like audio file uploads to S3 buckets, offer a compelling way to orchestrate audio processing workflows. Think of them as automated workers that spring into action whenever a new audio file arrives. This setup is particularly valuable for voice cloning, where tasks like transcription or audio manipulation are essential.
Imagine a scenario where you upload a new audio file for a voice cloning project. The upload triggers a Lambda function which can initiate a transcription process using Amazon Transcribe. This asynchronous approach, using async/await in the JavaScript code within Lambda, prevents delays and keeps the workflow flowing smoothly. Once the transcription is complete, another Lambda function could be triggered to further process the transcription data, possibly adding metadata or enhancing it with AI tools. The beauty of this architecture is its flexibility. You could expand this to process various types of audio files, such as WAV, MP3, or even audio streams, and tailor it to specific voice cloning tasks.
Setting up such a workflow involves configuring S3 buckets, defining event triggers linked to Lambda functions, and scripting the Lambda functions themselves. Using async/await becomes crucial when you need to handle multiple operations concurrently, such as loading a large audio file or performing extensive audio analysis. This asynchronous execution within Lambda minimizes the chance of blocking the execution environment and keeps your overall processing nimble.
One benefit of employing Lambda functions for audio processing is their ability to scale automatically. This is particularly advantageous for situations with unpredictable workload demands, for example, during the release of a new podcast or audiobook. When the load increases, Lambda dynamically provisions more resources to handle the requests. Conversely, when the demand subsides, Lambda automatically reduces resources, leading to cost optimization as you only pay for the compute time consumed.
Lambda functions can also improve cost efficiency in comparison to managing your own servers. You won't need to worry about infrastructure maintenance and scaling. However, we must keep in mind that while this might appear like a silver bullet, blindly embracing Lambda for every audio processing task can sometimes lead to unforeseen issues, including managing complex dependencies and security aspects. You also have to be aware of cold start times and error handling in certain situations.
While this serverless paradigm offers many advantages, it's also important to recognize the potential for errors within the audio processing chains themselves. Just as with any automation system, there are occasional hiccups. In these situations, having robust error-handling mechanisms within Lambda functions is crucial to prevent interruptions or data loss in critical parts of the audio workflow. Implementing proper logging and monitoring practices are also important for debugging and ensuring smooth operations.
Furthermore, the integration of Lambda with AWS Step Functions allows for building complex audio processing workflows involving multiple steps or branches. This feature enables sophisticated sequencing of tasks in your audio pipelines. A key advantage is that it enhances flexibility, allowing you to adapt workflows for diverse audio projects without extensive code rewriting. For instance, you might create one branch to handle audiobook transcriptions and another for podcast editing tasks.
Of course, it's essential to consider the potential limitations. It's crucial to design the Lambda functions with careful consideration of memory usage and execution time, as you could encounter performance constraints in complex workflows. But overall, Lambda functions, due to their versatility, provide a promising avenue for automating and managing audio processing workflows, particularly when applied to tasks such as voice cloning or enhancing audio book productions.
This serverless approach can also facilitate collaboration in audio production, especially across different geographic locations or devices. Teams can easily access and modify audio files stored in S3 and initiate processing tasks with Lambda, fostering a more efficient and distributed workflow in audio productions. Despite its power and potential, it's important to approach this with some level of critical analysis of the actual workflows in question. The goal is to use the proper tools for the job.
Streamlining Audio Processing Leveraging Async/Await in JavaScript for Efficient Voice Cloning Workflows - Optimizing Speech Synthesis with JavaScript Promises
Utilizing JavaScript Promises to optimize speech synthesis improves the efficiency and responsiveness of audio processing within applications like voice cloning, audiobook creation, and podcasting. The Speech Synthesis API allows developers to transform text into spoken audio, offering control over characteristics such as pitch, rate, and volume through the SpeechSynthesisUtterance object. Promises enable streamlined management of asynchronous operations related to speech synthesis, resulting in a smoother user experience by preventing the main thread from being blocked. This careful control over the audio output is particularly important when handling tasks requiring precision, such as smoothly integrating multiple audio clips or synchronized voices. Although, it's essential to recognize potential complexities, like appropriately managing the sequence of audio tasks and upholding audio quality consistently throughout the synthesis process. While Promises help streamline the process, these factors still require attentive planning and coding to ensure successful and high-quality audio outcomes.
The JavaScript Speech Synthesis API offers a compelling way to transform text into spoken words, enhancing the interactivity of web applications, particularly in areas like voice cloning and audio book production. We can control and manipulate these synthesized audio segments, referred to as "utterances," using the SpeechSynthesis interface of the Web Speech API. This interface provides methods to manage the synthesis process, including selecting available voices, and initiating, pausing, and halting speech.
However, dealing with the asynchronous nature of audio loading and manipulation within a web application can be tricky. Here's where JavaScript Promises come into play. By using promises, we can optimize the handling of these asynchronous tasks associated with speech synthesis, leading to a smoother and more user-friendly audio experience, especially critical in voice-cloning workflows where seamless transitions are essential. Moreover, the async/await syntax further enhances the management of these tasks, making the code more readable and manageable.
The JavaScript Text to Speech API is designed with scalability in mind. This is important for applications like audio book productions and podcast creation, where handling diverse audio formats and minimizing server load are crucial considerations. The SpeechSynthesisUtterance object gives us fine-grained control over the characteristics of the spoken audio, such as pitch, rate, and volume.
By incorporating the Speech Synthesis API into web applications, developers can introduce voice interactions into user experiences, both for capturing user input and providing audio output. It's noteworthy that the Web Speech API isn't solely about text-to-speech; it also incorporates speech recognition capabilities, further expanding its utility in voice-enabled services.
One challenge that arises when working with the API relates to playing multiple audio segments sequentially. Implementing a proper sequence in our code becomes essential to prevent unexpected behavior or jarring transitions. This is especially true in voice cloning applications, where glitches in audio playback can degrade the quality of the cloned voice. While we can achieve a level of control, careful sequencing is needed to ensure smooth transitions when managing multiple synthesized audio utterances.
While the Speech Synthesis API offers a solid foundation, it also presents some intricacies. Browser compatibility can be inconsistent, and achieving consistent results across platforms might involve extra development effort. Additionally, ensuring proper memory management, especially during extended use or with intensive audio processing, requires meticulous attention to detail. The API can be a bit temperamental, with edge cases related to handling specific audio configurations or managing timing nuances. It's a testament to the complexity of audio processing within the browser environment. However, if properly used, the Speech Synthesis API and careful handling of the asynchronous tasks within JavaScript hold significant potential for enriching the audio experiences provided by web applications.
Streamlining Audio Processing Leveraging Async/Await in JavaScript for Efficient Voice Cloning Workflows - Custom Audio Processors for Real-Time Voice Manipulation
The ability to manipulate audio in real-time, particularly voice, has become increasingly important, driving the development of custom audio processors. These processors are now essential for applications ranging from voice cloning to enhancing podcast production. They provide developers the freedom to design unique audio effects and dynamically adjust vocal characteristics, thereby improving the overall listening experience. The integration of tools like AudioWorklet, part of the Web Audio API, offers a significant advantage by moving computationally intensive audio processing away from the main thread. This offloading ensures smooth, low-latency audio streams, which is critical for a natural, seamless audio experience. This is especially valuable in applications like voice cloning where high-fidelity audio is paramount. While the power and flexibility of custom audio processors are undeniable, developers need to remain aware of the potential complications that come with this increased control. Striking a balance between sophisticated audio manipulation and manageable code implementation is key to success. There can be issues with consistency across browsers, and the potential for unexpected behavior when handling intricate audio workflows should not be overlooked.
The development of custom audio processors for real-time voice manipulation presents a fascinating realm of research and engineering challenges. These processors often rely on intricate digital signal processing techniques like phase vocoding or granular synthesis, allowing for adjustments to pitch, speed, and timbre while striving to maintain a natural-sounding voice. This is particularly vital when the goal is to generate convincingly realistic voice clones.
However, latency—the delay between audio input and output—is a constant concern in voice manipulation. Even small delays can disrupt the sense of synchronicity, especially when integrating with visual content. Asynchronous programming becomes crucial for handling the processing of multiple audio streams and preventing these latency-related issues.
Further complicating the picture is the creation of an audio effects chain. These effects, applied as a series of interconnected processing nodes, can each introduce latency. The collective impact of these nodes can become a performance bottleneck unless managed thoughtfully. Carefully optimizing audio flow within this chain is critical for achieving high-quality sound output.
While we can manipulate voice characteristics like pitch, accent, and intonation with these processors, achieving natural-sounding results remains a significant hurdle. Even subtle imperfections can make a synthetic voice sound robotic or artificial, highlighting the ongoing need for refinements in the algorithms.
One technique to accelerate processing and minimize resource usage is zero-copy buffering. By minimizing the copying of audio data as it passes through different processing stages, we can reduce CPU load and achieve quicker real-time processing. This is especially important for resource-intensive applications like live broadcasting.
Beyond static processing, dynamic effect application allows for live modification of voice modulation. This can be useful in podcasts and audiobooks for adding emphasis or adjusting tone based on the script, potentially enhancing the user's experience.
A key aspect of developing and debugging these custom processors is incorporating audio visualization capabilities. Asynchronous visualization techniques can provide immediate feedback, allowing developers to monitor spectral changes and levels, aiding in workflow debugging and ensuring proper spatial audio cues.
Furthermore, integrating multiple web APIs, such as the Web Audio API and the MediaStream API, becomes necessary in many audio processing workflows. This can greatly enhance the versatility of applications, allowing for simultaneous capturing and processing of live audio, essential for voice cloning and podcast production.
The issue of compression artifacts is also prominent in the domain of voice cloning. During audio transmission, whether online or through phone calls, compression algorithms can degrade the original voice quality. Addressing these artifacts during the cloning process becomes crucial to ensure the synthetic voice preserves the clarity and nuances of the original.
Lastly, the field of custom audio processors has significant potential for integration with machine learning. Neural networks can be used to analyze voice samples, which can ultimately lead to real-time, context-driven voice synthesis. This holds promise for fundamentally revolutionizing the way synthetic voices are generated for use in diverse applications such as audiobooks and voice interaction systems.
While we've made great strides in the realm of custom audio processing for real-time voice manipulation, continued research and development are essential to address the remaining challenges and unlock the full potential of this intriguing technology.
Get amazing AI audio voiceovers made for long-form content such as podcasts, presentations and social media. (Get started for free)
More Posts from clonemyvoice.io: